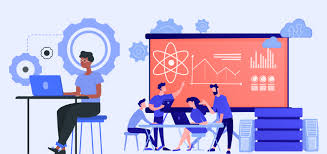
Managing State in React
What is State?
In React, state represents the data that a component needs to render and respond to user interactions. State is mutable and can be updated using the setState
method, triggering re-rendering of the component.
Using State in Functional Components
With the introduction of React hooks, functional components can now utilize state using the useState
hook.
- Initializing State:
- Updating State:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
You clicked {count} times
);
}
function Counter() {
const [count, setCount] = useState(0);
const increment = () => {
setCount((prevCount) => prevCount + 1);
};
return (
You clicked {count} times
);
}
State Management in Class Components
Prior to hooks, state was managed using class components. Class components have a state object and use this.setState
to update state.
- Initializing State:
import React, { Component } from 'react';
class Counter extends Component {
constructor(props) {
super(props);
this.state = {
count: 0,
};
}
render() {
return (
You clicked {this.state.count} times
);
}
}
State Management Best Practices
- Use State Sparingly: Keep state local to the component where it's needed to avoid unnecessary complexity.
- Lift State Up When Necessary: If multiple components need access to the same state, lift the state up to their closest common ancestor.
- Immutability: Always update state immutably, either by using the functional form of
setState
or by creating a new object/array. - Consider State Management Libraries: For complex applications, consider using state management libraries like Redux or React Context API.
Learning Resources
- React Documentation - State and Lifecycle: Refer to the official React documentation for comprehensive explanations and examples of state and lifecycle methods.
- freeCodeCamp React Projects: Build real-world projects to apply state management techniques and gain hands-on experience.